Demystifying JavaScript Hoisting: A Deep Dive
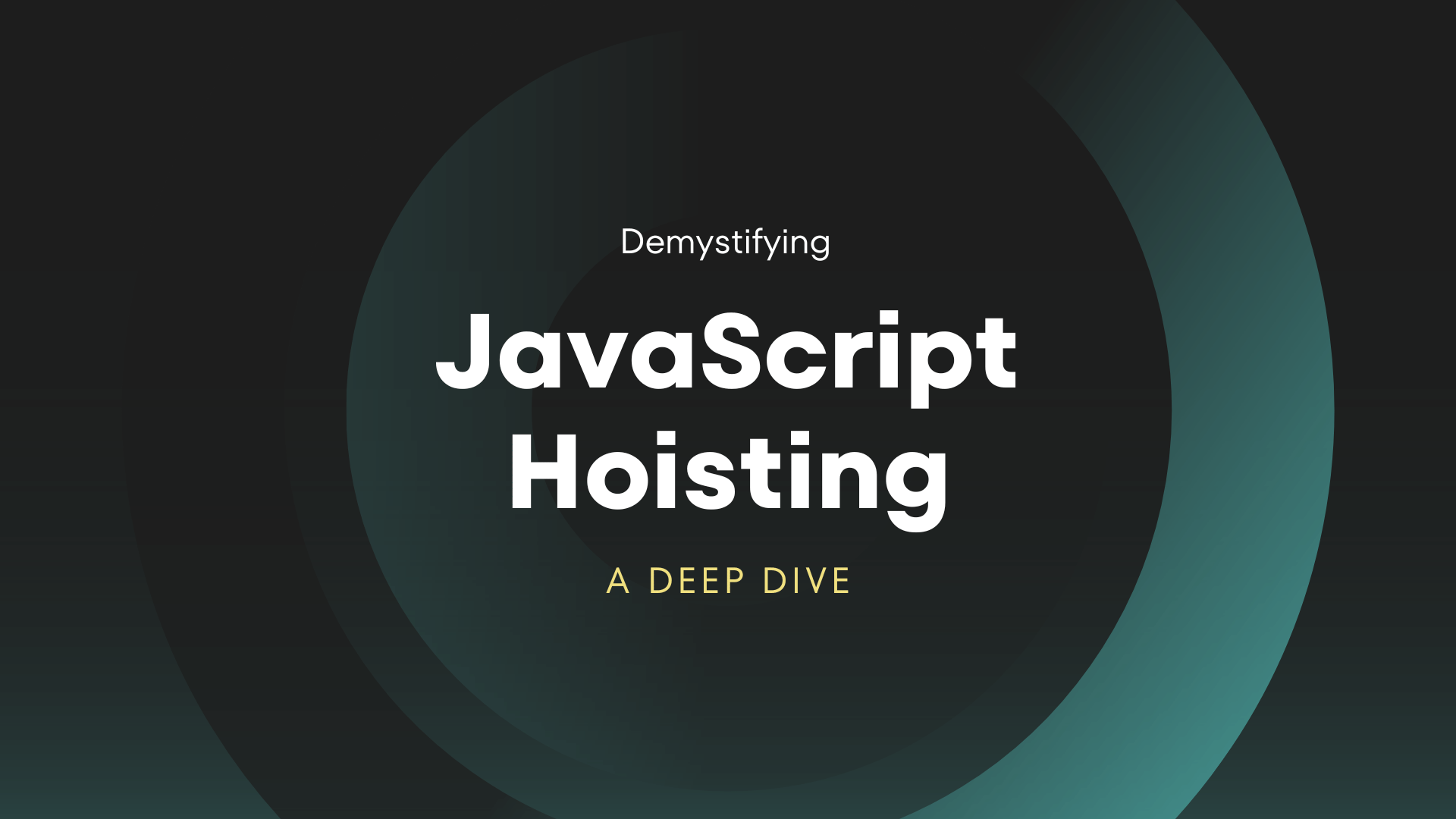
In JavaScript, ‘hoisting’ is a fundamental concept that often perplexes developers, especially those new to the language. It refers to the behavior where variables and function declarations are moved to the top of their containing scope during the compilation phase. This blog post aims to clarify this concept through explanations and code examples.
Understanding Hoisting
In JavaScript, before the code execution, the interpreter ‘hoists’ or ‘lifts’ variable and function declarations to the top of their respective scopes. This means that variables and functions can be used before they are declared in the code. However, it’s important to note that only declarations are hoisted, not initializations.
Variable Hoisting
Variables declared with var are hoisted to the top of their function or global scope, but their initialization is not. Let’s look at some examples.
Code Example: Variable Hoisting
1
2
3
console.log(myVar); // undefined
var myVar = 5;
console.log(myVar); // 5
In this example, myVar is hoisted to the top with a default initialization of undefined. It’s not until the actual assignment (var myVar = 5;) that it gets its value.
Function Hoisting
Function declarations are hoisted, which means they can be called before they are declared in the script.
Code Example: Function Hoisting
1
2
3
4
5
hoistedFunction(); // Outputs: "This function has been hoisted."
function hoistedFunction() {
console.log("This function has been hoisted.");
}
This function call works because hoistedFunction is hoisted to the top of its scope with its definition.
Hoisting in ES6 (let and const)
let and const declarations are also hoisted, but they maintain a temporal dead zone from the start of the block until the declaration is encountered. Accessing them before the declaration results in a ReferenceError.
Code Example: let and const Hoisting
1
2
3
4
5
console.log(myLetVar); // ReferenceError: myLetVar is not defined
let myLetVar = 3;
console.log(myConstVar); // ReferenceError: myConstVar is not defined
const myConstVar = 'constant';
These errors occur because let and const are in a temporal dead zone from the start of the block until the declaration is initialized.
Best Practices and Conclusion
While hoisting can be useful, it’s generally best to declare variables and functions at the top of your scope to avoid confusion and potential errors. Understanding hoisting helps in debugging and writing predictable JavaScript code.
As a JavaScript developer, being aware of how hoisting works under the hood is crucial. Remember, while hoisting brings declarations to the top, initializations are not hoisted. Always declare variables and functions before using them to keep your code clean and error-free.
Understanding hoisting is just one step in mastering JavaScript, but it’s a significant one. Keep exploring and experimenting, and you’ll gain a strong command over these fundamental concepts.
Additional References
Hoisting - W3Schools
Hoisting - MDN Web Docs
Best wishes in your coding journey!